How to Create a Chat App in Android: A Comprehensive Guide
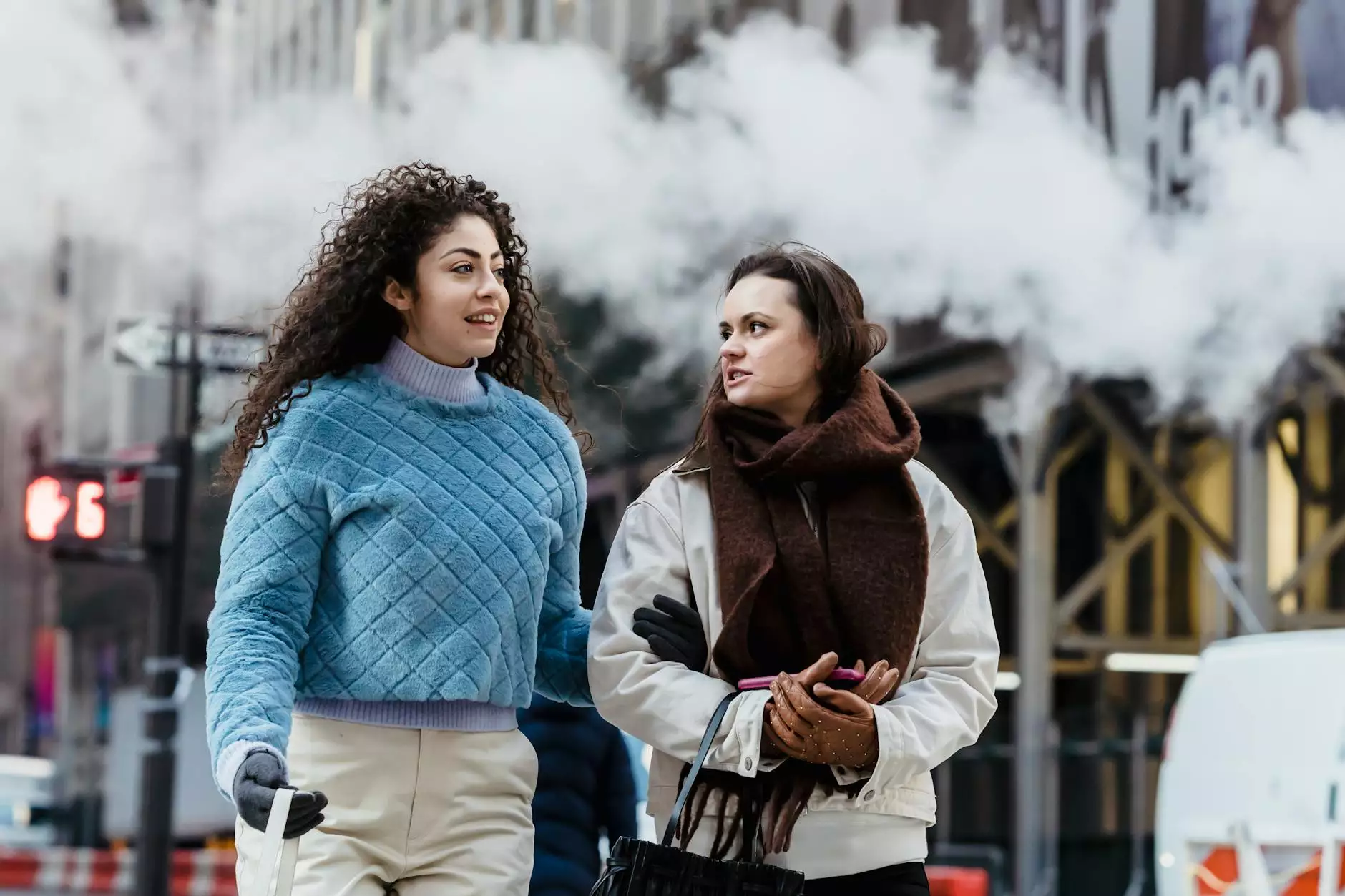
Building a chat application for Android can be an exciting venture, especially given the increasing demand for real-time communication platforms. In this extensive guide, we will explore how to create a chat app in Android, detailing every necessary step from initial concept to deployment. Whether you're a novice developer or an experienced programmer, this article will provide insights and techniques to help you craft a successful messaging application.
Understanding the Basics of Chat Applications
Before delving into the actual development process, it's crucial to grasp the fundamental concepts of a chat application. Chat apps typically involve the following core functionalities:
- User Authentication: Secure login and registration systems.
- Real-Time Messaging: Instant delivery and receipt of messages.
- Message Storage: Mechanisms for storing chats on the server.
- Push Notifications: Alerts for new messages and updates.
- User Profiles: Customizable profiles for users.
These features form the backbone of any chat application, and mastering them is key to building a successful product.
Choosing the Right Tools for Development
The next step in how to create a chat app in Android involves selecting the appropriate tools and technologies. The choices you make will significantly influence the development process and the app’s performance. Below are essential components you should consider:
1. Programming Language
For Android development, the primary programming languages are Java and Kotlin. While Java has been the traditional choice, Kotlin offers modern syntax and features that enhance productivity. We recommend using Kotlin for a smooth development experience.
2. Development Environment
Android Studio is the official Integrated Development Environment (IDE) for Android app development. It provides all the necessary tools to help streamline your development process.
3. Backend Servers
A chat application requires a robust backend to manage users, messages, and other complex functionalities. Popular options include:
- Firebase: A complete mobile app development platform that provides cloud-based real-time database and authentication services.
- Node.js: A server-side JavaScript framework ideal for handling asynchronous communication.
- PHP and MySQL: A traditional but effective stack for many developers.
Each of these options comes with unique advantages, so choose one that fits your project scope best.
Setting Up Your Android Project
Once you've selected your tools, the next step is to set up your Android project. Follow these steps:
- Open Android Studio and start a new project.
- Select the appropriate template (e.g., Empty Activity).
- Configure your project settings, including the project name and package name.
- Set the minimum API level based on your target audience.
These configurations will lay the groundwork for your development journey.
Implementing User Authentication
To maintain security and track individual messages, you'll need to implement user authentication. Firebase Authentication is a robust choice for this purpose. Here’s how you can set it up:
- Create a Firebase project on the Firebase Console.
- Enable Firebase Authentication and choose your preferred sign-in providers (e.g., Email/Password, Google, etc.).
- Integrate the Firebase SDK into your Android project by adding dependencies in the `build.gradle` file.
- Build login and registration activities using the appropriate Firebase methods to handle user credentials.
With user authentication in place, your application now has a secure entry point.
Creating the Chat Interface
The user interface is crucial in a chat application. You want it to be intuitive and engaging. Here’s how to structure your chat UI:
1. Layout Design
Using XML, design the chat screens. A typical chat interface includes:
- A RecyclerView for displaying messages.
- Input field for users to type and send messages.
- Send button to transmit messages.
2. Message Adapter
Create an adapter class for your RecyclerView to manage message display. Each message can be represented as a separate view.
3. Message Model
Define a model class for messages that includes attributes like sender ID, message text, and timestamp. This organization will help maintain clean code.
Implementing Real-Time Messaging
Real-time messaging is the heart of any chat application. We will demonstrate how to achieve this with Firebase Realtime Database:
- Structure your database to store messages under a unique chat room ID.
- Use FirebaseDatabase to listen to message updates and fetch new messages.
- Upon sending a message, write it to the database and update the RecyclerView accordingly.
This setup ensures messages are delivered instantly, creating a responsive chat experience.
Adding Push Notifications
To keep users informed of new messages, integrating push notifications is essential. You can use Firebase Cloud Messaging (FCM) for this purpose. Here’s a brief overview of the process:
- Set up FCM in your Firebase project.
- Request user permissions for notifications on their devices.
- Trigger notifications when a new message is added to the Firebase Realtime Database.
- Implement a service to handle incoming notifications and display them to users.
Testing Your Application
Once you've built your chat app, rigorous testing is necessary to ensure functionality and performance. Consider these testing strategies:
- Unit Testing: Write tests for individual components to verify their behavior.
- Integration Testing: Test how different parts of the app work together, particularly user authentication and message sending.
- Usability Testing: Gather feedback from real users to identify areas for improvement.
Utilizing a testing framework like JUnit or Espresso can enhance your testing workflow significantly.
Deploying Your Chat App
Before you can share your chat application with the world, you have to deploy it. Follow these steps to publish your app on the Google Play Store:
- Prepare your app for release by removing debug code and optimizing performance.
- Generate a signed APK or App Bundle using Android Studio.
- Create a developer account on the Google Play Console.
- Submit your app along with all required information, including a description, screenshots, and category.
After review and approval from Google, your app will be available for users to download!
Future Enhancements
This guide provided a foundational understanding of how to create a chat app in Android. However, you can take your application further by considering additional features:
- End-to-End Encryption: Ensures user privacy and security.
- File Sharing: Allow users to send images, videos, and documents.
- Group Chats: Enable communication among multiple users.
- Typing Indicators: Notify users when someone is typing.
These enhancements add significant value and can distinguish your app in a crowded marketplace.
Conclusion
Creating a chat application for Android is an enriching journey that combines creativity and technical prowess. By following this guide and leveraging the right tools and frameworks, you can build a functional and engaging chat app that meets users’ needs. Embrace the learning curve, continuously optimize your application, and keep exploring new features to stay ahead in the competitive market.
For more resources and tools tailored to mobile app development, be sure to visit nandbox.com, where you'll find additional insights and support for your software development projects.